3 minutes
Add a Map to Your Android App With Mapbox and Kotlin
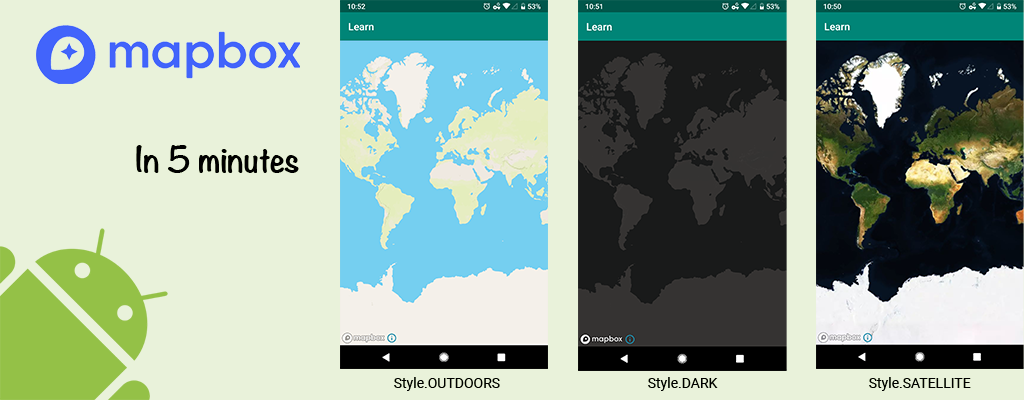
There's a lot of reasons you'd want to use MapBox over Google Maps for your next project. Good news, it's a simple process. You can get a map un and running in a few minutes and the API mirrors a the Google Maps SDK, so switching from one to the other shouldn't be too much pain.
Let’s get started
First thing first, you need to get an Access Token from MapBox:
-
Head to MapBox website and get an access token. This only takes a minute and you will need to create an account.
-
Next, name your app and click on Create Token. You’ll see a lot of settings in there. For now, you don’t need any of them so just leave them as is.
-
Upon creation, you can retrieve your token on the next page. Keep ahold of this, we’ll need it very soon.
Just show me the code already
Adding MapBox to your Android app is really simple and requires little boilerplate. Let’s start by just showing a map on your screen. If you’re using a Fragment
, call mapview.onDestroy()
in onDestroyView()
instead of onDestroy()
.
**/app/build.gradle** (Check for latest version here) ```json implementation 'com.mapbox.mapboxsdk:mapbox-android-sdk:8.0.0' ```
/res/values/strings.xml
<string name="access_token">your_access_token</string>
/res/layouts/activity_map.xml
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:mapbox="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.mapbox.mapboxsdk.maps.MapView
android:id="@+id/mapview"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</RelativeLayout>
/src/…/MapActivity.kt
class MapActivity: AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// This goes before setContentView. You can also place it in your Application class
Mapbox.getInstance(this, getString(R.string.access_token))
setContentView(R.layout.activity_map)
mapview.onCreate(savedInstanceState)
// Initializing is asynchrounous- getMapAsync will return a map
mapview.getMapAsync { map ->
// Set one of the many styles available
map.setStyle(Style.OUTDOORS) { style ->
// Style.MAPBOX_STREETS | Style.SATELLITE etc...
}
}
}
public override fun onStart() {
super.onStart()
mapview.onStart()
}
public override fun onResume() {
super.onResume()
mapview.onResume()
}
public override fun onPause() {
super.onPause()
mapview.onPause()
}
public override fun onStop() {
super.onStop()
mapview.onStop()
}
override fun onLowMemory() {
super.onLowMemory()
mapview.onLowMemory()
}
override fun onDestroy() {
super.onDestroy()
mapview.onDestroy()
}
override fun onSaveInstanceState(outState: Bundle) {
super.onSaveInstanceState(outState)
mapview.onSaveInstanceState(outState)
}
}
And that’s it! Your activity should display a map. Next, you’ll want to customize it in your xml or using the map
object you retrieved in getMapAsync { }
. In order to do so, I’ll take you through a few common usecases. Keep reading!
Customize your map
Notice we provided the xmlns:mapbox
namespace in our xml? This is because it is one way you can customize your maps. Here are some examples
<com.mapbox.mapboxsdk.maps.MapView
android:id="@+id/mapview"
android:layout_width="match_parent"
android:layout_height="match_parent"
mapbox:mapbox_cameraZoom="12"
mapbox:mapbox_cameraTargetLat="37.7749"
mapbox:mapbox_cameraTargetLng="-122.4194"
mapbox:mapbox_cameraTilt="50"
/>
You can also apply the same parameters through your Kotlin code, which can be a better choice when you want these settings to be dynamic.
map.moveCamera(
CameraUpdateFactory.newLatLngZoom(LatLng(37.7749, -122.4194), 12.0)
)
And here is what the result looks like:
445 Words
2022-07-27 12:34 +0000